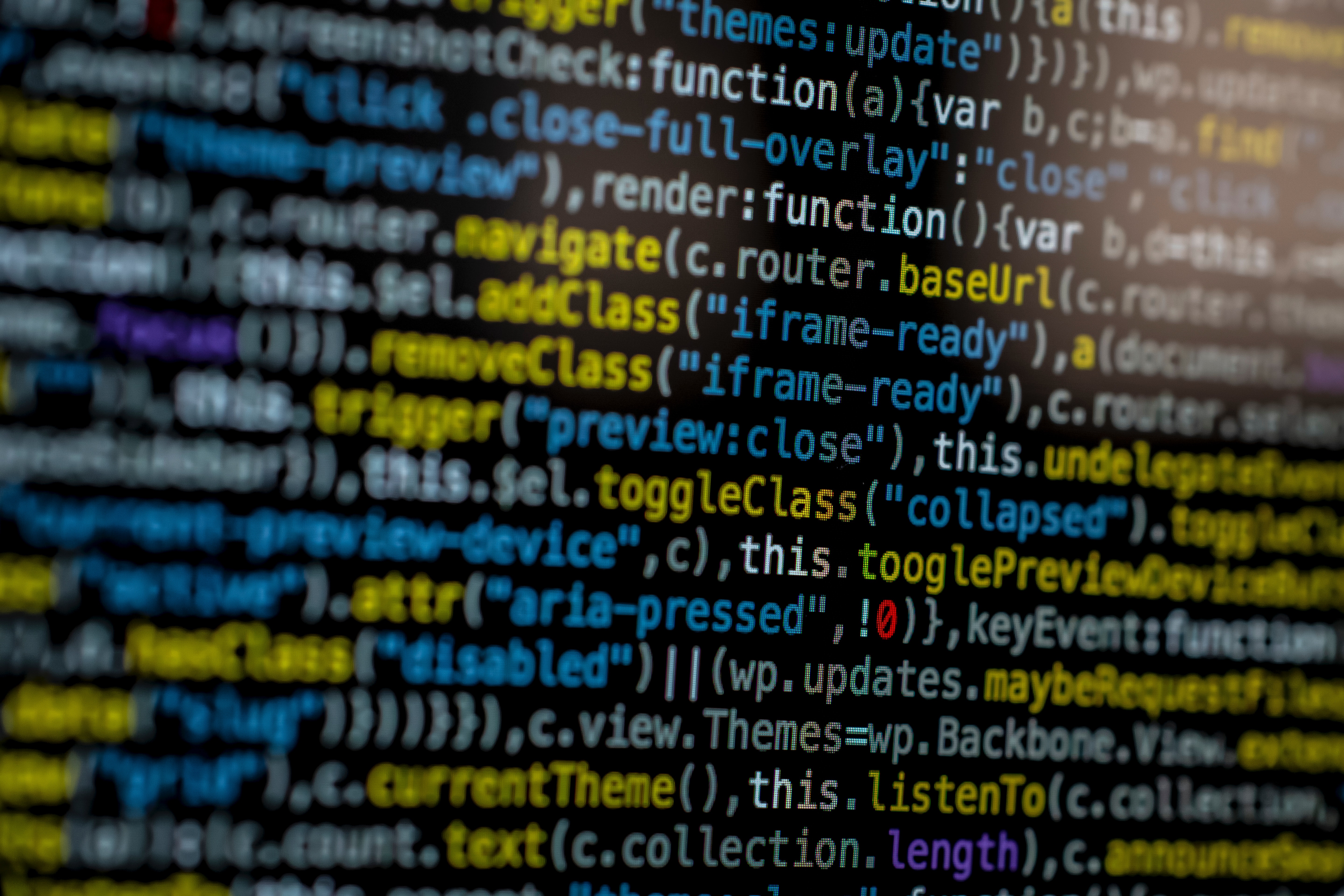
JavaScript Tutorial #5 - A Simple ToDo List
What We Cover
- Query Selector
- Events
- For Loops (learn more)
- Arrays (learn more)
- Functions
In this tutorial we'll build a simple To-Do list. Once you've done a task on your list, simply click the task and watch it disappear. This is a fun little exercise that you can customize to do a lot more than just be a simple list. This will give you practice with creating functions, using arrays, and running a for loop.
See the Pen Untitled by Justin (@zadees) on CodePen.
HTML
<html>
<head>
<title>My To-Do List</title>
</head>
<body>
<h1>My To-Do List</h1>
<form id="form">
<input type="text" id="input" placeholder="Enter something to do">
<button type="submit">Add</button>
</form>
<ul id="list"></ul>
</body>
</html>
JavaScript
// Get a reference to the form and input elements
const form = document.querySelector("#form");
const input = document.querySelector("#input");
let todos = []; // create an empty array to store our todos
let listItems = []; // HTML list items for our list
const addItem = (item) => {
if(item){
listItems += `<li class="task" id="${todos.length}">${item}</li>`;
}
}
const updateList = () => {
listItems = "";
for(item of todos){
listItems += `<li class="task" id="${todos.length}">${item}</li>`;
}
// Add the new items to the list
document.getElementById("list").innerHTML = listItems;
}
// Remove a task from the to-do list
const removeTask = (id, item) => {
// create a new array
const newList = [];
// loop through the todos and only add tasks that weren't removed to the new array
for(task of todos) {
if(task != item){
newList.push(task);
}
}
todos = newList; // assign the new list to the todos
updateList();
}
const list = document.querySelector('ul');
// Listen for the clicks on the todo list
list.addEventListener("click", (event) => {
removeTask(event.target.id, event.target.innerText);
});
// Listen for the form's submit event
form.addEventListener("submit", (event) => {
// Prevent the default form submission behavior and keep the page from reloading
event.preventDefault();
// Get the value of the input field
const item = input.value;
// Create a new list item
todos.push(item);
// Updates the list items
addItem(item);
// Add the new item to the list
document.getElementById("list").innerHTML = listItems;
// Clear the input field
input.value = "";
});