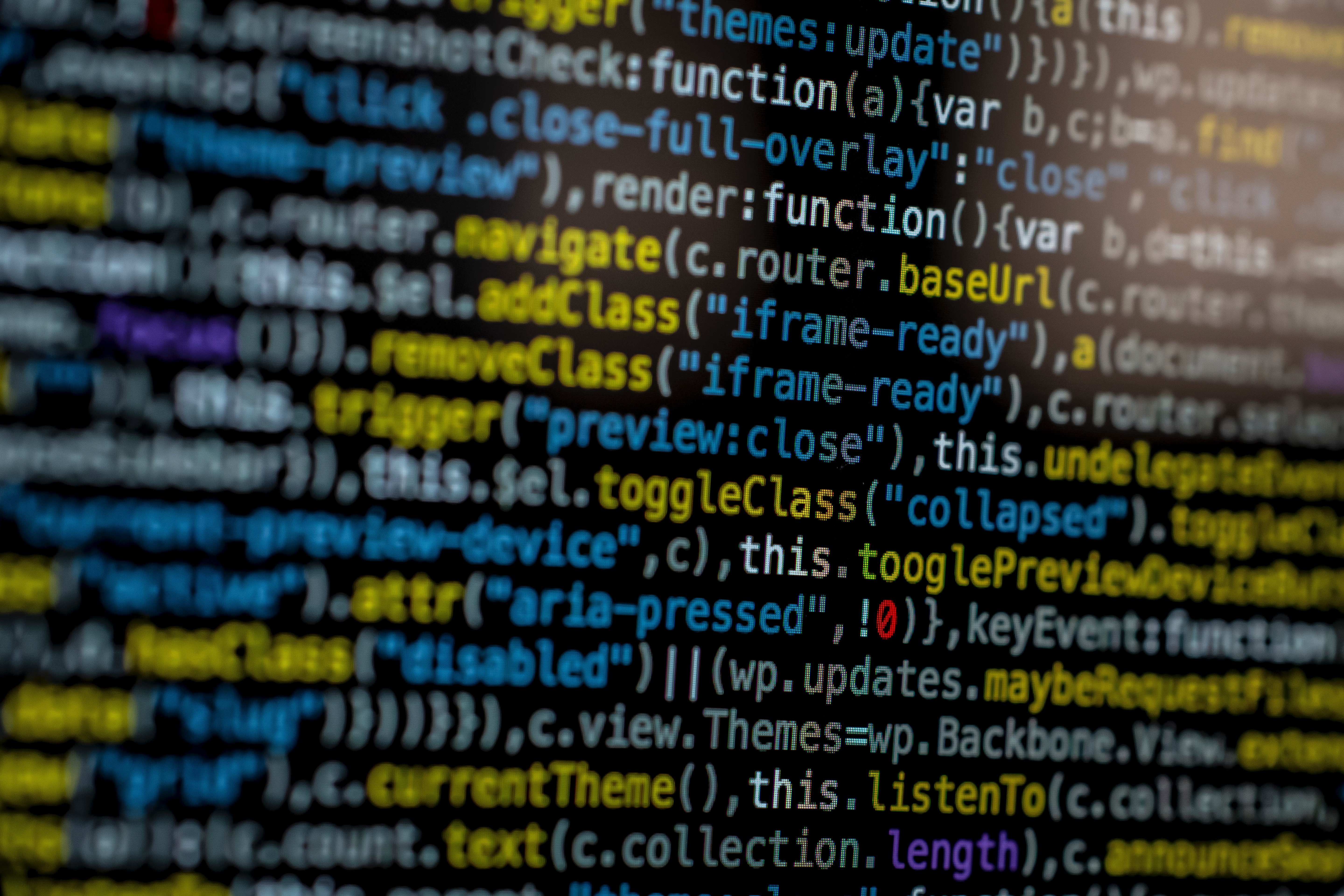
Arrays
In JavaScript, an array is a data type that is used to store a collection of elements. Each element in the array can be accessed by a numeric index, which is a number that represents the position of the element in the array. For example, the first element in the array is at index 0, the second element is at index 1, and so on.
Arrays in JavaScript are a powerful tool for storing and manipulating data, and they are a fundamental part of the language. You can use arrays to store any data type, including numbers, strings, objects, and even other arrays.
Here is an example of how you might create an array in JavaScript:
const myArray = []; // defines an empty array
// adds the text John to the first value of an array
myArray[0] = 'John';
console.log(myArray); // Outputs ["John"]
// adds the text Smith to the end of the array
myArray.push('Smith');
console.log(myArray); // Outputs ["John","Smith"]
// adds the text Bella to the end of the array
myArray.push('Bella');
console.log(myArray); // Outputs ["John","Smith","Bella"]
// Sort the array ascending order
console.log(myArray.sort()); // Outputs ["Bella","John","Smith"]
// Reverse the order of the array
console.log(myArray.reverse()); // Outputs ["Smith","John","Bella"]
// View the length of the array (number of items)
console.log(myArray.length) // Outputs 3