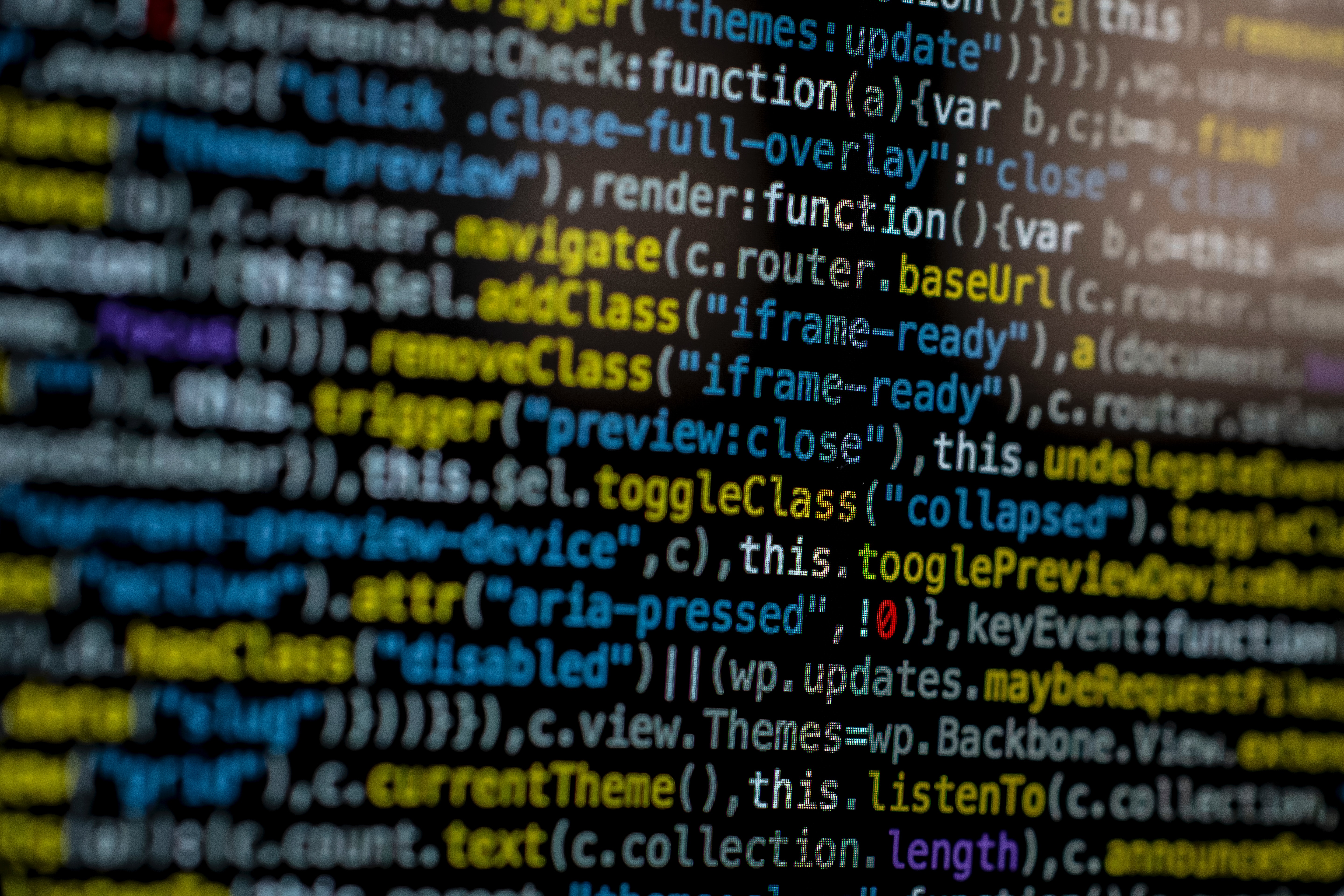
For Loop
The For Loop
In JavaScript, the for
loop is a control flow statement that allows you to execute a block of code a certain number of times. It consists of three parts: the loop initialization, the loop condition, and the loop iteration. The loop initialization is where you initialize the variables that will be used in the loop. The loop condition is a boolean expression that determines whether the loop will continue to run. If the condition is true, the code inside the loop will be executed, and then the iteration statement will be executed to update the state of the loop. The loop will continue to run as long as the condition remains true. Here is an example of a for
loop in JavaScript:
for (var i = 0; i < 5; i++) {
console.log("Hello, world!");
}
// OUTPUT
// "Hello, world!"
// "Hello, world!"
// "Hello, world!"
// "Hello, world!"
// "Hello, world!"
In this example, the for
loop will execute the code inside the loop 5 times, with the variable i
starting at 0 and increasing by 1 each time the loop runs. On the first iteration, i
will be 0, on the second iteration it will be 1, and so on. The loop will stop running when i
reaches 5, because the loop condition (i < 5
) will be false.
The For Of Loop
In JavaScript, the for of
loop that loops over iterable data structures like Arrays. In this example we will loop through an array of user objects. We'll show you the traditional for
loop vs the for of
loop.
For Loop
const users = [
{ name: 'John', age: 27 },
{ name: 'Sarah', age: 30 },
{ name: 'Maria', age: 43 }
];
// loop through the users and log out a message
for(let i = 0; i < users.length; i++){
const message = `User ${users[i].name} is ${users[i].age} years old.`;
console.log(message);
}
// OUTPUT
// "User John is 27 years old."
// "User Sarah is 30 years old."
// "User Maria is 43 years old."
For Of Loop
const users = [
{ name: 'John', age: 27 },
{ name: 'Sarah', age: 30 },
{ name: 'Maria', age: 43 }
];
// loop through the users and log out a message
for(let user of users){
const message = `User ${user.name} is ${user.age} years old.`;
console.log(message);
}
// OUTPUT
// "User John is 27 years old."
// "User Sarah is 30 years old."
// "User Maria is 43 years old."
While both of these loops do the same thing, the cleaner approach of the for of
loop makes it cleaner when working with long blocks of code. Neither of these is wrong, so feel free to use whichever concept you feel best works for you.
The For In Loop
The for in
loop is different in that it loops through the properties of an Object instead of items in an iterable data structure. We'll do our same example as above and when building our message we'll use the for in
loop to loop through the user object.
const user = { name: 'John', age: 27, job: 'Janitor' };
// loop through the users and log out a message
for(let x in user){
const message = `${x} - ${user[x]}`;
console.log(message);
}
// OUTPUT
// "name - John"
// "age - 27"
// "job - Janitor"