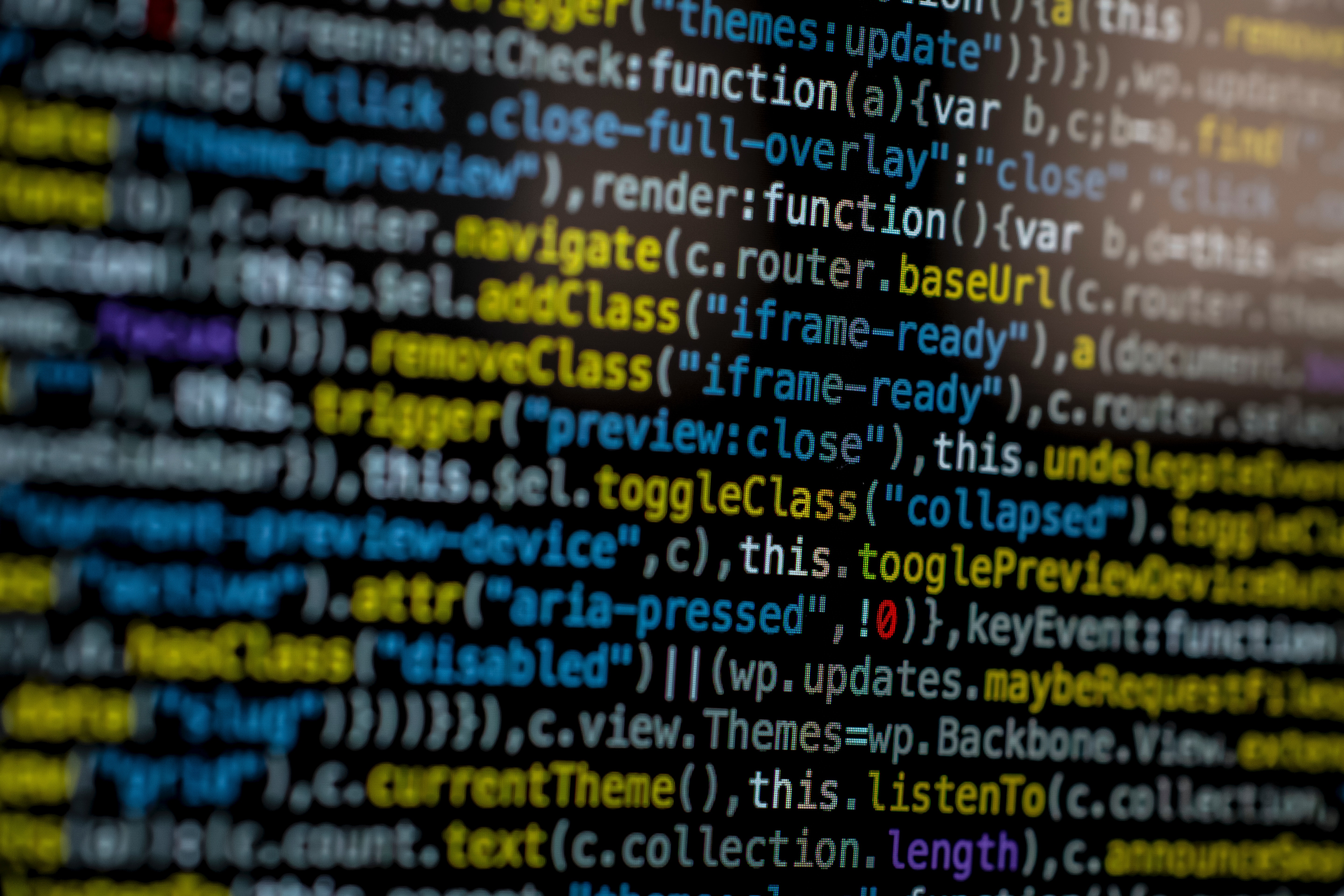
JavaScript Tutorial #3 - Variables
What We Cover
What Is A Variable?
In JavaScript, a variable is a named container that stores a value. Variables are used to store data that can be accessed and modified by different parts of your program.
To create a variable in JavaScript, you use the var, const, or let
keyword followed by the variable name, like this:
var myVariable;
You can then assign a value to the variable using the =
operator, like this:
myVariable = "Hello World";
You can also declare the variable and assign it a value in a single step, like this:
var myVariable = "Hello World";
To use a variable in your code, simply reference its name wherever you want to use its value. For example:
console.log(myVariable); // Outputs "Hello World"
Naming A Variable
When naming a variable it is important to choose a name that clearly defines the data you are storing. Let's say we are looking to store a user's name. We would want to define our variable like this var userName = "John Smith";
This way, whenever you are referencing the variable, you will know what the variable is supposed to be representing.
Typically when writing in JavaScript we have a convention that we name variables starting with a lowercase letter. Then each word after is capitalized. This is referred to as Camel Case.
An example of this would be a variable defining an user's first name. var userFirstName = "John";
When To use const, let, and var When Declaring a Variable
const
, let
, and var
are three different ways to declare variables. Each of these has its own specific characteristics and uses, so it's important to understand the differences between them.
var
The var
keyword is the oldest dway to declare a variable in JavaScript. Variables declared with var
are either function-scoped or global-scoped, depending on where they are declared. This means that a var
variable is accessible from anywhere within the current function, or from anywhere in the global scope if it's declared outside of a function.
One of the key differences between var
and the other two keywords is that var
variables are hoisted to the top of the current scope. This means that you can reference a var
variable before it's declared in your code and you can reference it outside of the function that it was defined in. For example:
if (true) { var myVar = "Hello World"; console.log(myVar); // Outputs "Hello World" } console.log(myVar + '!'); // Outputs "Hello World!"
let
The let
keyword was introduced in the ES6 version of JavaScript, and it provides a new way to declare variables. Unlike var
variables, let
variables are block-scoped, which means that they are only accessible from within the block in which they are declared. For example:
if (true) {
let myVar = "Hello World";
console.log(myVar); // Outputs "Hello World"
}
console.log(myVar + '!'); // ReferenceError: myVar is not defined
Notice how when we change the var in the code before to let when declaring myVar it continues to output "Hello World", but when it tries to call the variable outside of the if function, it gives us a ReferenceError.
This block-scoping behavior of let
variables can make your code easier to read and understand, because you can clearly see the boundaries within which a let
variable can be accessed. It can also prevent errors caused by accidentally referencing a variable from outside of its intended scope.
Another advantage of let
variables is that they are not hoisted to the top of the current scope like var
variables are. This means that you must declare a let
variable before you can reference it in your code, which can help prevent accidental referencing of uninitialized variables.
Additionally, let
variables are re-assignable, which means that you can change their value after they are declared. This is in contrast to const
variables, which are immutable and cannot be re-assigned once they are declared. This makes let
variables more flexible than const
variables in certain situations.
In summary, the benefits of using let
instead of var
in JavaScript include block-scoping, lack of hoisting, re-assignability, and improved readability and maintainability of your code.
const
The const
keyword is also a new addition to JavaScript, and it was introduced in the same version as let
. const
variables are similar to let
variables in that they are block-scoped, but there is one key difference: const
variables are immutable, which means that their value cannot be changed once they are assigned.
For example:
const myVar = "Hello World";
myVar = "Goodbye World"; // TypeError: Assignment to constant variable
he code above, the myVar
variable is declared using the const
keyword and assigned the value "Hello World"
. If you try to reassign a new value to myVar
, you will get a TypeError
because const
variables are immutable.
Conclusion
In summary, the key differences between const
, let
, and var
are as follows:
var
variables are function- or global-scoped and are hoisted to the top of the current scope.let
variables are block-scoped and are not hoisted.const
variables are block-scoped, are not hoisted, and are immutable.