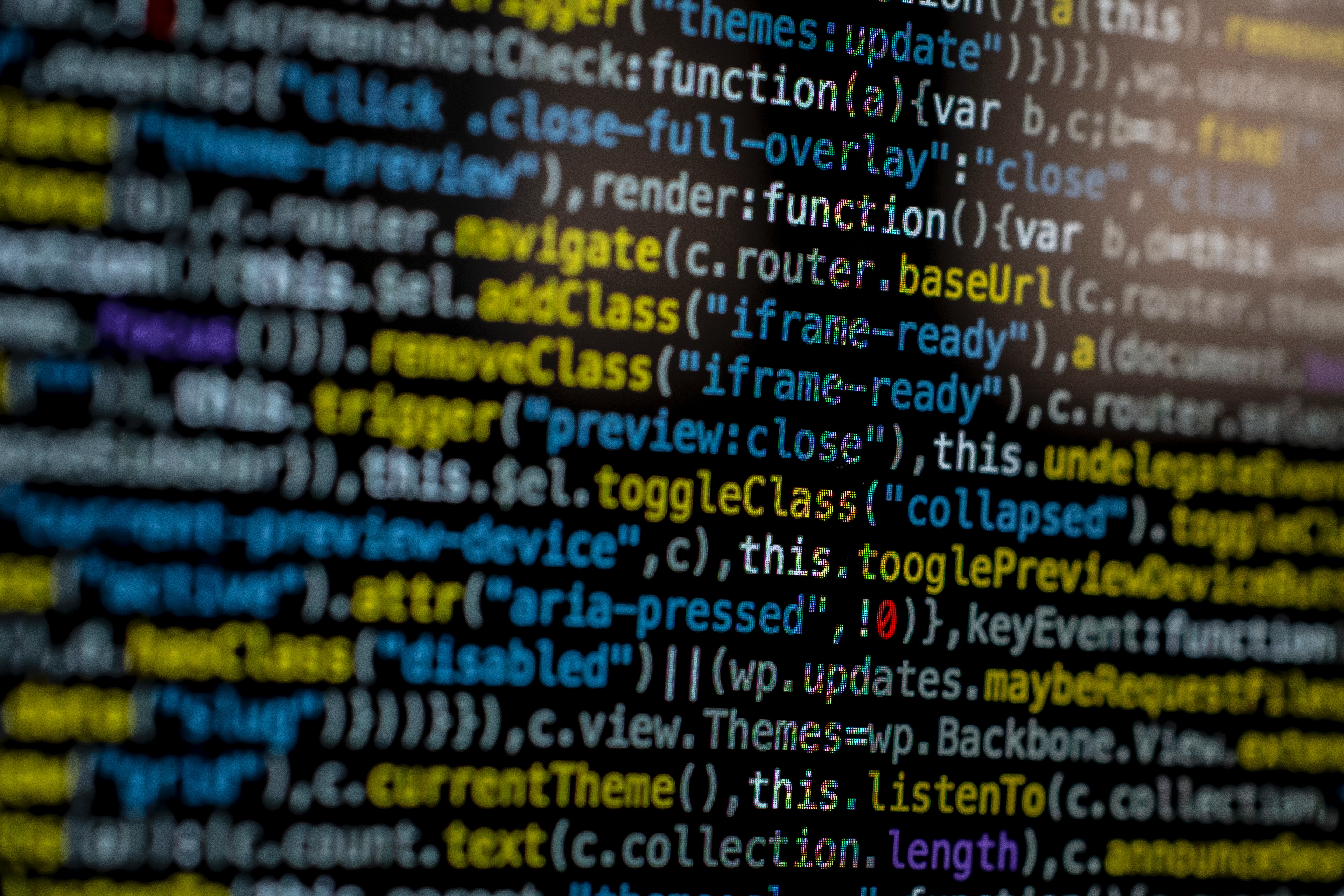
JavaScript Tutorial #4 - A Simple Calculator
What We Cover
- Math Operations
- Event Listeners
- Variables
- Functions
Here's a simple JavaScript calculator app that allows the user to perform basic mathematical operations such as addition, subtraction, multiplication, and division. Below is the full code that we are planning on using. This will give you an HTML form that you can use to build a GUI (Graphical User Interface) to interact with once the code is written.
The BEST way to learn to code is to find something you want to create and then break it down into simple tasks. A great idea here would be to just create the functions and test them out by calling them directly in the JavaScript code. Once you know they are working correctly, then move on to adding HTML code and getting a single button to work calling a function. After that you should be able to quickly get everything else to work. Once you learn to think about how to break things down into small components, then you will be able to really master coding. Learning syntax is useful, but it isn't a great way to learn a language.
What We're Building
See the Pen JavaScript Tutorial #4 - A Simple Calculator by Justin (@zadees) on CodePen.
HTML
<input type="text" id="operand1" />
<br /><br />
<input type="text" id="operand2" />
<br /><br />
<div>Result: <span id="result"></span></div>
<br />
<button id="add">Add</button>
<button id="subtract">Subtract</button>
<button id="multiply">Multiply</button>
<button id="divide">Divide</button>
JavaScript
// Set our variables and get a reference to all of the HTML elements
const addButton = document.getElementById('add');
const divideButton = document.getElementById('divide');
const multiplyButton = document.getElementById('multiply');
const subtractButton = document.getElementById('subtract');
const result = document.getElementById('result');
const operand1 = document.getElementById('operand1');
const operand2 = document.getElementById('operand2');
// Define a function to add two numbers
const add = (x, y) => {
return +x + +y;
}
// Define a function to subtract two numbers
const subtract = (x, y) => {
return +x - +y;
}
// Define a function to multiply two numbers
const multiply = (x, y) => {
return +x * +y;
}
// Define a function to divide two numbers
const divide = (x, y) => {
return +x / +y;
}
// Listen for a click on the Add button
addButton.addEventListener("click", () => {
result.innerHTML = add(operand1.value,operand2.value);
});
// Listen for a click on the Subtract button
subtractButton.addEventListener("click", () => {
result.innerHTML = subtract(operand1.value,operand2.value);
});
// Listen for a click on the Multiply button
multiplyButton.addEventListener("click", () => {
result.innerHTML = multiply(operand1.value,operand2.value).toFixed(2);
});
// Listen for a click on the Divide button
divideButton.addEventListener("click", () => {
result.innerHTML = divide(operand1.value,operand2.value).toFixed(2);
});
Once we run this code we will get a simple calculator that takes two numbers and performs the operation on them. Let's review a few of the things here that you may not be aware of.
- Comments - The
//
lines are ignored by JavaScript and are used for commenting a single line of code. If you would like to comment more than a single line the multi-line comment begins with/*
and ends with*/
. This will allow you to comment out as much code as you want. - Functions - Functions are a powerful and versatile feature of JavaScript that allow you to write modular and reusable code. unction is a block of code that can be called by other parts of your program. Functions allow you to define a reusable piece of code that can be executed whenever you need to perform a specific task. Inside the function, you can include any code that you want to be executed when the function is called. For example, in the add function we defined a function that performs a mathematical operation on two numbers (x and y) and returns the result. These two numbers that are passed into the function are called parameters and are often referred to as params.
- Events - In this example we are using the
addEventListener
and specifically looking for theclick
event. Once a user clicks on that element, then we will call a function. In this case, there are no parameters so the function is noted as() => { }
. This is the new ES6 way of using functions and replaces the old syntax offunction () { }
. - toFixed - You'll notice in the multiplyButton and the divideButton eventListener functions, that we append a
.toFixed(2)
after the function calls. This takes the result that is given, and ensures that it will be no longer than 2 decimal places. Try dividing 5 by 3 without the.toFixed(2)
to see the difference. - What's with the '+' sign in front of x and y? - When doing math on variables you want to ensure that they are all numbers prior performing the mathematical action. The + in front of a variable lets JavaScript know to treat this as a number and not a text string. Try removing the +'s in front of the x and the y in the
add
function. Now when you add 3 and 5 instead of getting 8, you will get 35. The reason is it looks at 3 and 5 as text and concatenates them together instead of actually adding them as numbers.
Now you have a simple calculator. Can you think of something else that you could build using what you learned in this tutorial? The best way to learn is by finding projects to work on and attempting to code them. Tutorials are great to get you started, but you'll learn more with practice. So try to find ways to build something creative with what you have learned so far. You can do this!