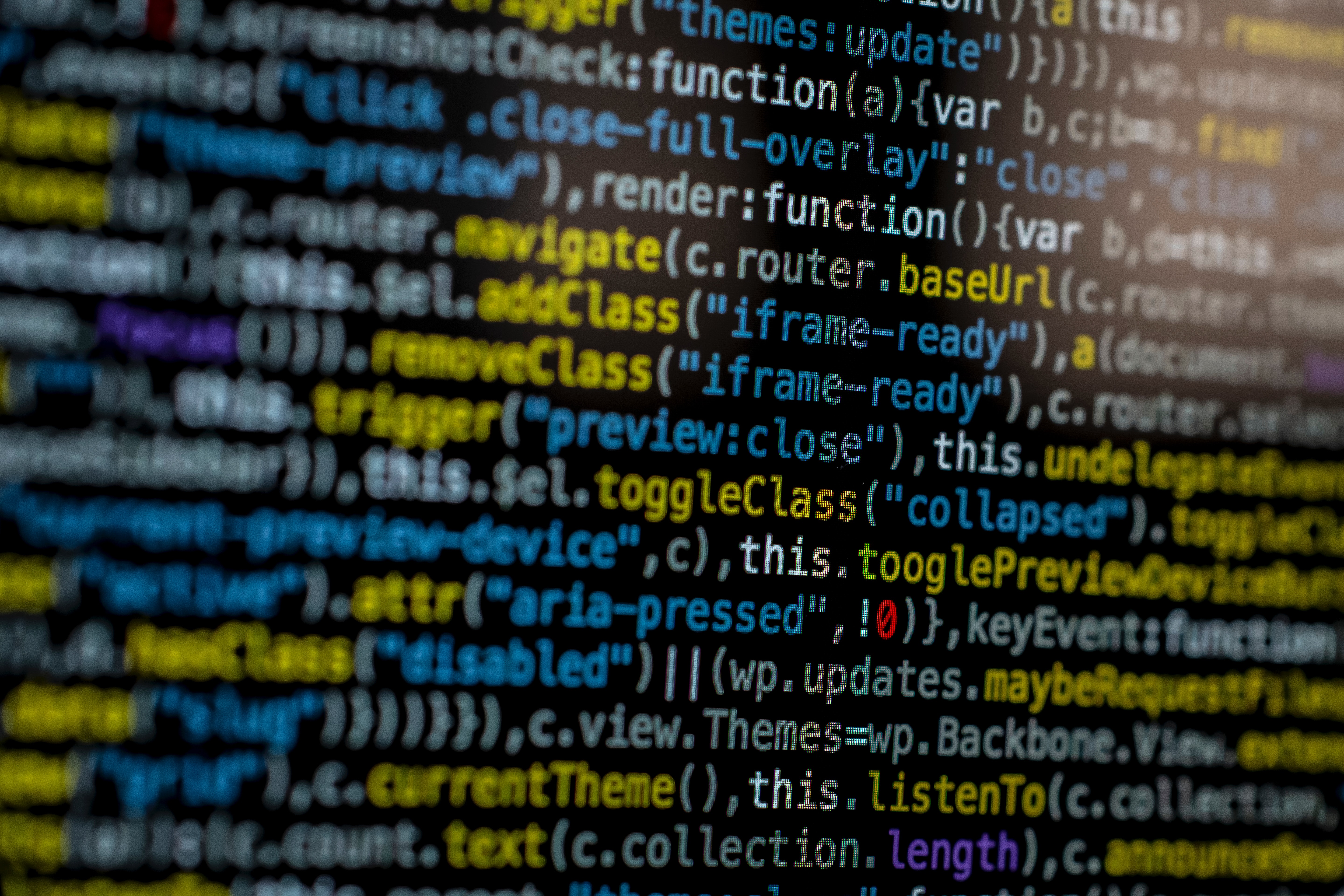
JavaScript Tutorial #2 - Output
What We Cover
- Logging out data to view in your console with a
console.log()
- Writing data to an HTML document using
document.write()
- Changing the contents of an HTML element using
innerHTML
- Showing an Alert box using
window.alert()
Console.log()
the console.log
function is a very useful tool for printing messages to the console in JavaScript. It can be used to print out any value or expression, including strings, numbers, booleans, and objects. Here's how to use it:
Open the JavaScript console in your browser. In most browsers, you can do this by pressing
F12
on your keyboard, or by right-clicking on the page and selecting "Inspect" from the context menu. If you are using Codepen, click the Console tab at the bottom of the screen.In your JavaScript code, use the
console.log
function to print out the value or expression you want to see in the console. There's an example below.- When you run your code, the message "Hello, world!" should appear in the console.
console.log("Hello, world!");
The console.log
function is very useful for debugging your code, as it allows you to see what's happening at different points in your program. You can use it to print out the values of variables, the results of expressions, and more, to help you understand how your code is working.
Below are a few more examples of how to use the console.log
function. Don't worry about understanding the code just yet, we'll go through that in later tutorials.
- Log the value of a variable:
let name = "Alice";
console.log(name); // prints "Alice"
- Log the result of an expression:
let x = 10;
let y = 20;
console.log(x + y); // prints 30
- Log the value of an object property:
let person = {
name: "Bob",
age: 30};
console.log(person.name); // prints "Bob"
document.write()
The document.write
function is a way to write content to an HTML document using JavaScript. It can be used to insert text, HTML elements, or other content into the page at the current location of the script. Here's how to use it:
In your JavaScript code, use the
document.write
function to write the content you want to add to the page.When you run your code, the message "Hello, world!" should be added to the page at the location where the script is executed.
document.write("Hello, world!");
It's important to note that the document.write
function should only be used for testing or debugging purposes, as it can cause problems if used improperly. For example, if you use it after the page has already finished loading, it will overwrite the entire page, replacing all of the existing content with the new content you wrote.
It's generally better to use other methods to add content to a page using JavaScript, such as using the innerHTML
property or creating elements using the document.createElement
method. These methods are more versatile and less likely to cause problems. Let me know if you have any other questions.
innerHTML
The innerHTML
property is a way to access and modify the contents of an HTML element using JavaScript. It allows you to read and write the HTML content within an element, including any text, HTML tags, and other elements. Here's how to use it:
In your JavaScript code, use the
document.getElementById
method to access the element you want to modify. This method takes theid
attribute of the element as its argument, and returns a reference to the element. Here's an example:HTML
<div id="app">Starting Data</div>
JavaScript
let myDiv = document.getElementById("app");
- Once you have a reference to the element, you can use the
innerHTML
property to read or write the content of the element. To read the content, simply access theinnerHTML
property of the element. For example:let content = myDiv.innerHTML;
console.log(content); // logs out: Starting Data To write new content to the element, simply assign a new value to the
innerHTML
property. For example:myDiv.innerHTML = "Hello, world!";
This will replace the existing content of the element with the new content you wrote. You can also use this property to add new content to the existing content, by concatenating the new content with the existing content. For example:
myDiv.innerHTML += "<p>This is some more data.</p>";
Notice the
+=
instead of the equals sign. This will add a newp
element to the end of the content ofmyDiv
. instead of just replacing it.
window.alert()
Thewindow.alert
function is a way to display a message to the user in a pop-up window using JavaScript. It can be used to alert the user to important information, to confirm an action, or to prompt the user for input. Here's how to use it:
If you are using codepen it will update after every time you make a change and can fire this blocking alert box while you are working. So, the best thing to do is to comment out your code until you are ready to use it. // window.alert("Hello, world!");
The //
before your window.alert makes the code a comment and it won't fire. When you are ready to test, remove the //
and your code will run.
In your JavaScript code, use the
window.alert
function to display the message you want to show to the user. Here's an example:window.alert("Hello, world!");
When you run your code, a small window should appear with the message "Hello, world!" in it. The window will have an "OK" button that the user can click to close it.
The window.alert
function is a very simple and easy way to display a message to the user, but it has some limitations. For example, it only allows you to display a single line of text, and it blocks the rest of the code from executing until the user clicks the "OK" button.
Here are a few more examples of how to use the window.alert
function:
Display a variable:
let name = "Alice";
window.alert("Hello, " + name + "!");Display the result of an expression:
let x = 10;
let y = 20;
window.alert(x + y);Display a message and get user input:
let name = window.prompt("Please enter your name:"); window.alert("Hello, " + name + "!");
The window.alert
function is a useful tool for displaying simple messages to the user, but it should be used sparingly, as it can be interruptive and annoying if overused.