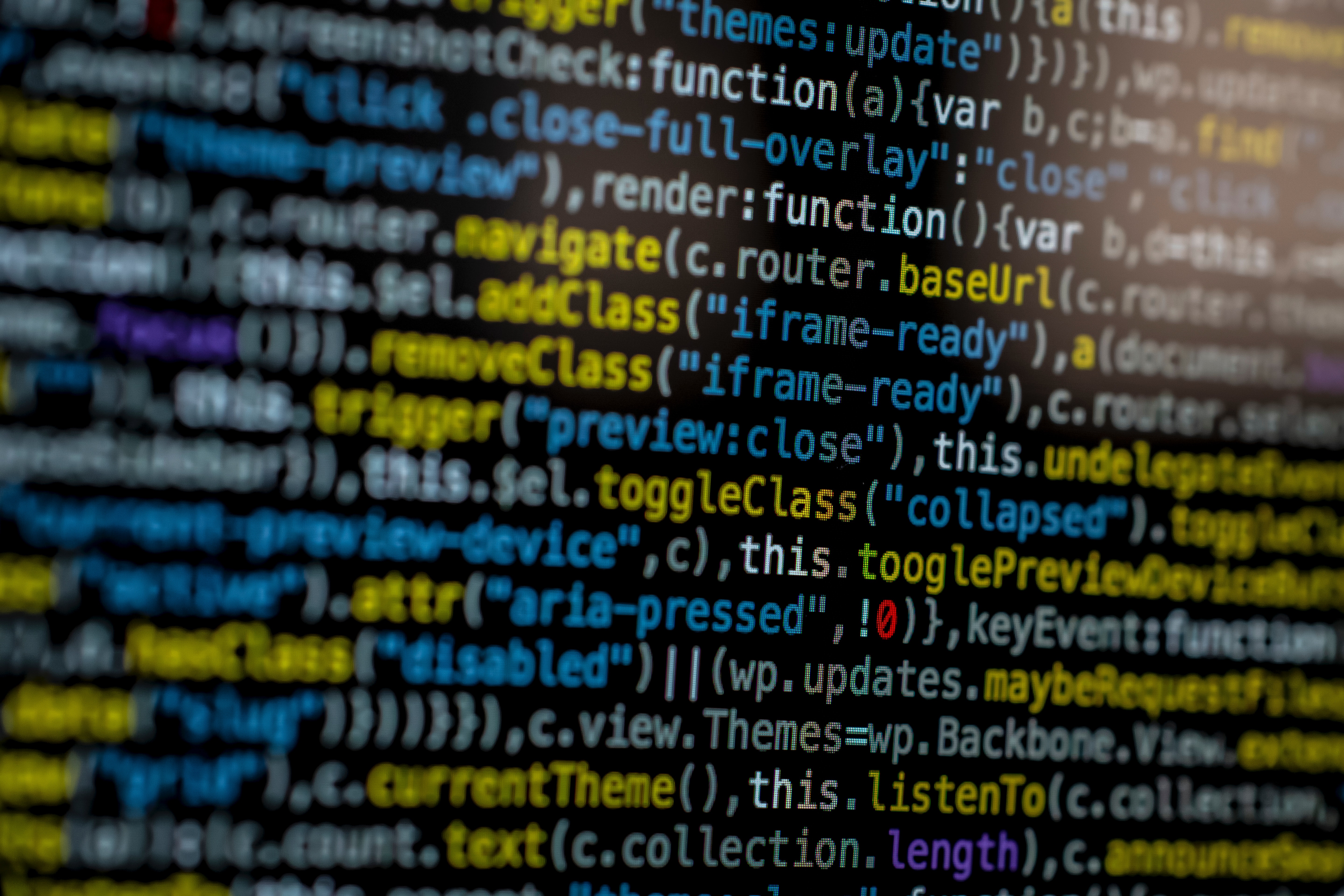
JavaScript Tutorial #1 - Intro To Javascript
JavaScript is a powerful programming language that allows you to add dynamic and interactive elements to your website. In this tutorial, we will focus on how to use JavaScript to create outputs, such as text and graphics, on your web page.
A quick way to get started is to use a site called codepen.io. Here you will have different sections to write both HTML and Javscript code.
To create an output with JavaScript, you first need to have a web page set up with the necessary HTML and CSS elements. Then, you can use the document.write()
function to add output to your web page.
For example, to create a simple text output, you can use the following code:
JavaScript
document.write("Hello, world!");
This will output the text "Hello, world!" on your web page.
You can also use the document.write()
function to create more complex outputs, such as tables and graphics. For example, to create a table with three rows and two columns, you can use the following code:
JavaScript
var tableData = `<table border="1" cellpadding="15">
<tr><td>Row 1, Column 1</td><td>Row 1, Column 2</td></tr>
<tr><td>Row 2, Column 1</td><td>Row 2, Column 2</td></tr>
<tr><td>Row 3, Column 1</td><td>Row 3, Column 2</td></tr>
</table>`;
document.write(tableData);
Output
Row 1, Column 1 | Row 1, Column 2 |
Row 2, Column 1 | Row 2, Column 2 |
Row 3, Column 1 | Row 3, Column 2 |
This will create a table on your web page with the specified text in each cell.
In addition to the document.write()
function, you can also use other JavaScript functions and libraries, such as the console.log()
function and the Canvas API, to create outputs on your web page.
Let's create a simple graphic using the Canvas API. We need an HTML Canvas element to start drawing on. You can either write that into your HTML code or use a document.write()
function as we did below. You can use the following code:
JavaScript
document.write("<canvas id='myCanvas'></div>");
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.fillStyle = "red";
ctx.fillRect(20, 20, 150, 100);
Output
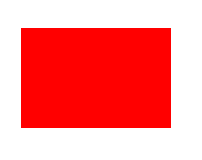
This code will create a red rectangle on your web page using the Canvas API.
In conclusion, JavaScript allows you to create a wide range of outputs on your web page, including text, tables, and graphics. By using the document.write()
function and other JavaScript functions and libraries, you can add dynamic and interactive elements to your website. We'll go into more detail in the next tutorials, this was mostly just to get a quick glimpse at the JavaScript language.