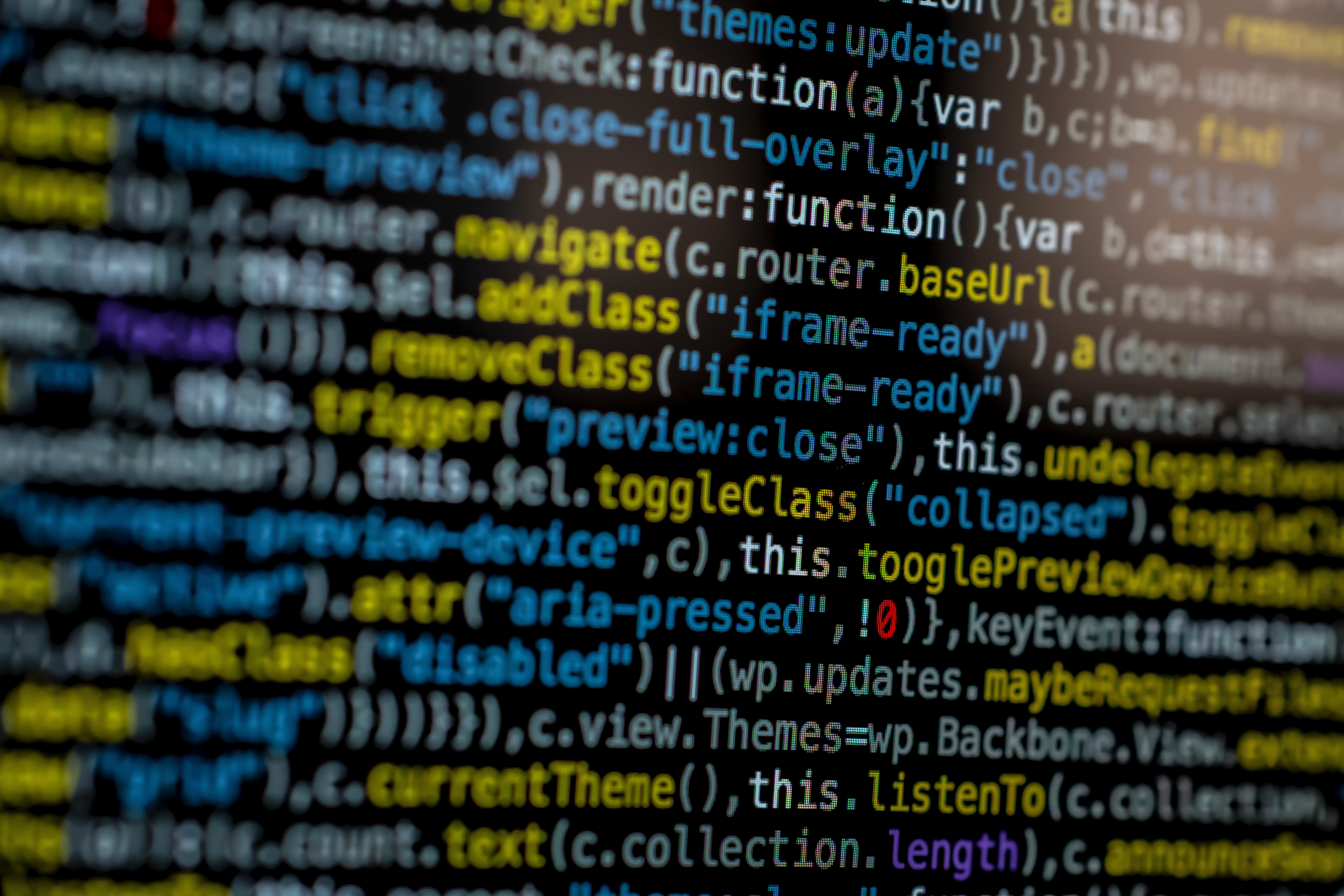
Functions
Functions are a powerful and versatile feature of JavaScript that allow you to write modular and reusable code. A function is a block of code that can be called by other parts of your program. Functions allow you to define a reusable piece of code that can be executed whenever you need to perform a specific task. Inside the function, you can include any code that you want to be executed when the function is called. For example, in the code below we take two strings and concatenate them together with a space between them. These two strings that are passed into the function are called parameters and are often referred to as params. In the example below, we named the parameters param1, and param2.
const myFunction = (param1, param2) => {
return `${param1} ${param2}`;
}
console.log(myFunction('Hello','World')); // Outputs "Hello World"
console.log(myFunction('John','Smith')); // Outputs "John Smith"
A function has a return
statement that will cause the function to stop executing. So any code you place after a return function will never execute. When a function is called the return statement will send back any values. In the example above you will see the return printed out to the console.