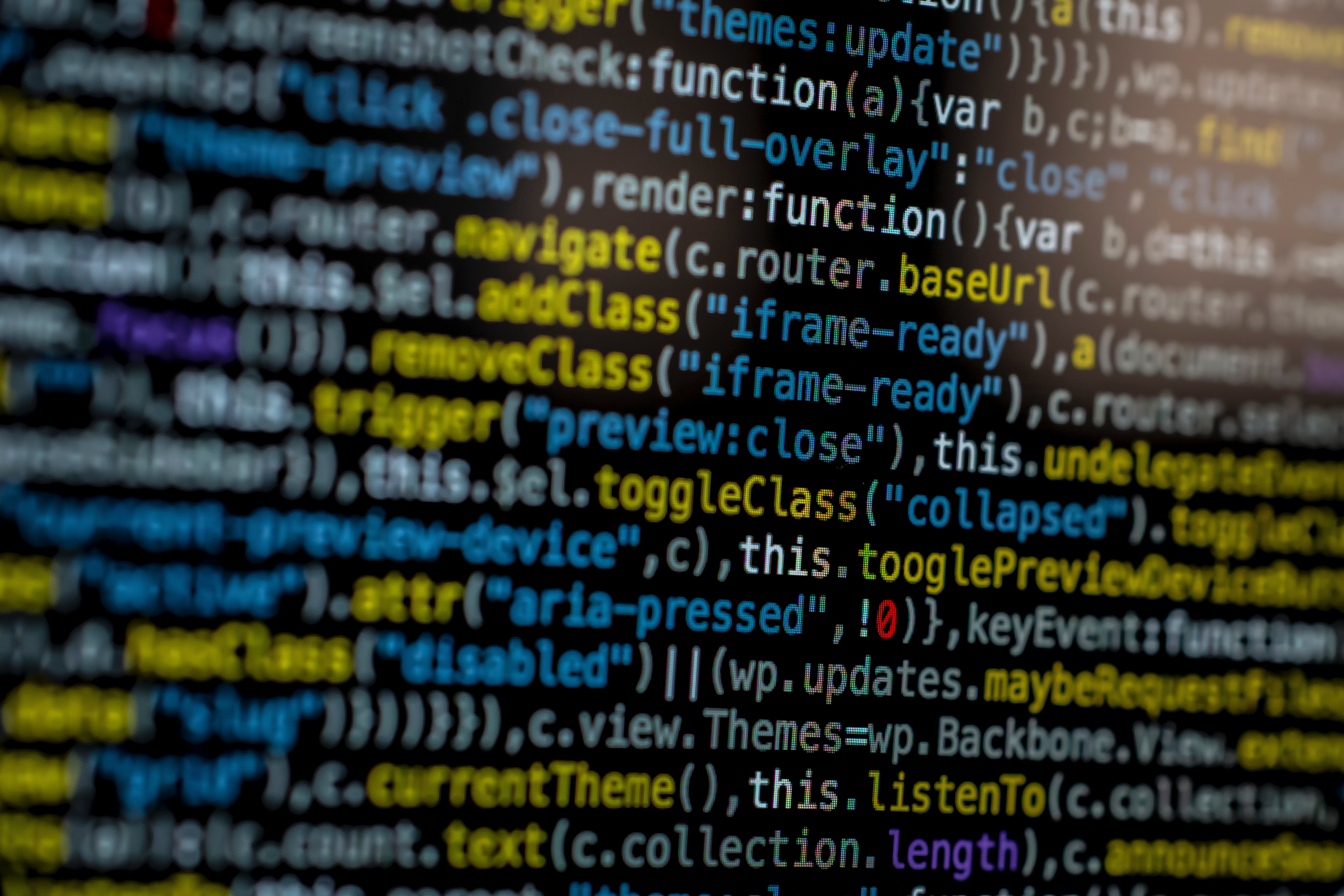
Strings
In JavaScript, a string is a sequence of characters enclosed in single or double quotes. For example, you could create a string like this:
let myString = "Hello, world!";
Once you have a string, you can use various methods and properties to manipulate it. For example, you can use the length
property to find out how many characters are in a string:
let myString = "Hello, world!";
console.log(myString.length); // This will output 13
There are a number of methods available on the String object that allow you to manipulate strings in various ways. Some of the most commonly used string methods are:
charAt()
: This method returns the character at a specific index in a string.concat()
: This method concatenates (joins) two or more strings together.indexOf()
: This method returns the index of the first occurrence of a specified substring within a string.lastIndexOf()
: This method returns the index of the last occurrence of a specified substring within a string.replace()
: This method replaces a specified substring with another substring.slice()
: This method extracts a part of a string and returns a new string.split()
: This method splits a string into an array of substrings based on a specified separator.toLowerCase()
: This method converts a string to lowercase letters.toUpperCase()
: This method converts a string to uppercase letters.
// charAt() example
const str = "Hello, world!";
console.log(str.charAt(0)); // Output: "H"
// concat() example
const str1 = "Hello, ";
const str2 = "world!";
console.log(str1.concat(str2)); // Output: "Hello, world!"
// indexOf() example
const str = "Hello, world!";
console.log(str.indexOf("world")); // Output: 7
// lastIndexOf() example
const str = "Hello, world! Hello, world!";
console.log(str.lastIndexOf("world")); // Output: 18
// replace() example
const str = "Hello, world!";
console.log(str.replace("world", "there")); // Output: "Hello, there!"
// slice() example
const str = "Hello, world!";
console.log(str.slice(7, 12)); // Output: "world"
// split() example
const str = "Hello, world!";
console.log(str.split(" ")); // Output: ["Hello,", "world!"]
// toLowerCase() example
const str = "Hello, world!";
console.log(str.toLowerCase()); // Output: "hello, world!"
// toUpperCase() example
const str = "Hello, world!";
console.log(str.toUpperCase()); // Output: "HELLO, WORLD!"
These are just some of the most commonly used string methods in JavaScript. There are many other useful string methods available, and you can learn more about them by reading the documentation or checking out other tutorials online.